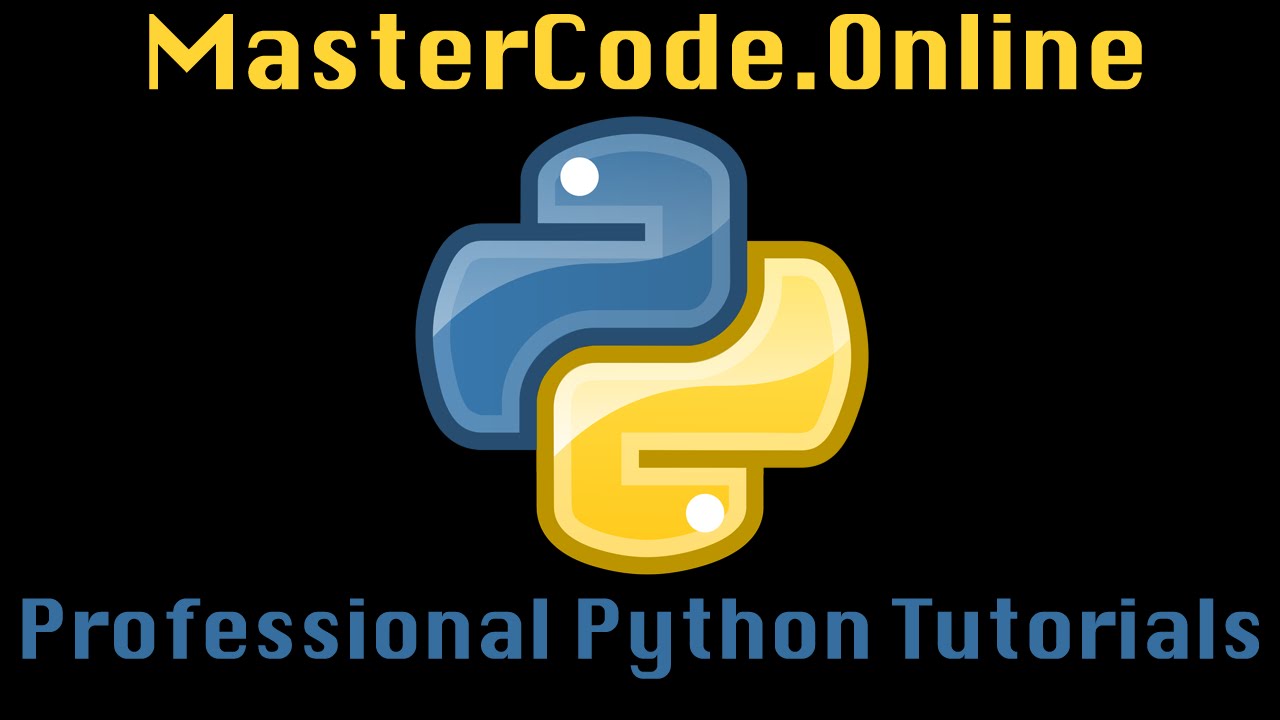
=======================================
Channel - https://goo.gl/pnKLqE
Playlist For This Tutorial - https://goo.gl/EyZFti
Latest Video - https://goo.gl/atWRkF
Facebook - https://www.facebook.com/mastercodeon...
Twitter - https://twitter.com/mastercodeonlin?l...
Website - http://mastercode.online
======================================
Python Find String Method
In this Python tutorial, we are going to look at the Python find string method. The find string method gives us the ability to find certain character or characters in a string object. If the character or characters is found then Python will return an index position of the first occurrence. If the search character or characters is not found the Python will return a -1 which indicates there was no match. Important to point out one last time that the find string method only returns a position. If you would like to know if content exists in a string object then use the in operator. More on the in operator in a later tutorial.
Python Find String Method Syntax
'string'.find('search string', start index, stop index)
Search String - Search string is required and must be in a string format.
Start Index - The start index is not required. Default start index in 0. If the stop index is present then the start index must be present.
Stop Index - The stop index is not required. Default is the end of the string.
Examples Of The Find String Method
Example 1:
a = 'New String'
Example 2:
a.find('e')
1
Example 3:
a.find('ring')
6
Example 4:
a.find('n')
8
Example 5:
a.find('s')
-1
Example 6:
a.find('w', 1)
2
Example 7:
a.find('t', 2, 5)
-1
Examples Explained
Example 1:
a = 'New String' - We create a new string object and which will be represented by the variable 'a'.
Example 2:
a.find('e') - In this example of the find string method we call our string object via the variable 'a' and call the find string method on our string object. We provide an argument of 'e' to the find string method.
1- We are returned 1 which indicates that the find string method found a 'e' at 1 index position. Note if there is other 'e' in the string object the find string method will on return the first occurrence in the object.
Example 3:
a.find('ring')- In this example of the find string method we call our string object via the variable 'a' and call the find string method on our string object. We provide an argument of 'ring' to the find string method.
6- We are returned 6 which indicates the location of start to the substring that was found.
Example 4:
a.find('n')- In this example of the find string method we call our string object via the variable 'a' and call the find string method on our string object. We provide an argument of 'n' to the find string method.
8 - We are returned '8' because the find string method is case sensitive and the first 'N' is capitalized the find string method skips the 'N' and continues till it finds the next 'n' and returns 8.
Example 5:
a.find('s')- In this example of the find string method we call our string object via the variable 'a' and call the find string method on our string object. We provide an argument of 's' to the find string method.
-1 - We are returned '-1' because the find method did not find any lower case 's'. -1 means that the find string method has not found any match.
Example 6:
a.find('w', 1)- In this example of the find string method we call our string object via variable 'a' and call the find string method on our string object. We provide an argument of 'w' to the find string method and a starting index position of 1.
2- We are returned 2 because the find string method found a match for the second index spot.
Example 7:
a.find('t', 2, 5)- In this example of the find string method we call our string object via variable 'a' and call the find string method on our string object. We provide an argument of 't' to the find string method and a starting index of 2 and ending index of 5.
-1 - We are returned -1 because the find string method did not find any matches.
Conclusion
In this Python tutorial we looked at the Python find string method. If you have any questions about this string method leave a comment below.
Python Find String Method | |
17,282 views views | 12,868 followers |
112 Likes | 112 Dislikes |
Education | Upload TimePublished on 24 Sep 2015 |
No comments:
Post a Comment